1. Row:
Row là một widget được sử dụng để hiển thị các child widget theo chiều ngang. Mặc định Row widget không thể scroll được. Nếu bạn có một dòng widget và muốn chúng có thể scroll nếu không đủ chỗ, hãy xem xét sử dụng ListView Class.
Nếu chúng ta muốn hiển thị ba widget trong một hàng, chúng ta có thể tạo một Row widget như dưới đây:
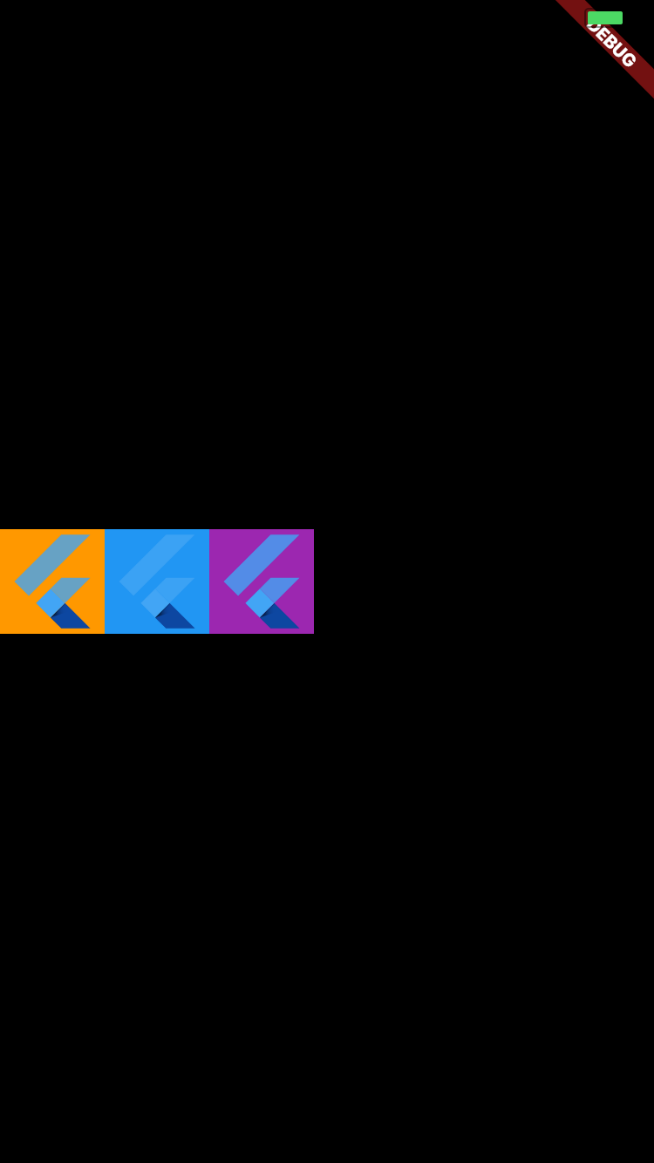
2. Column
Cột là một widget được sử dụng để hiển thị các child widget theo chiều dọc. Mặc định Column widget không scroll được. Nếu bạn có một cột widget và muốn chúng có thể scroll nếu chiều cao thiết bị không đủ, hãy xem xét sử dụng ListView.
Nếu chúng ta muốn hiển thị ba widget trong một cột, chúng ta có thể tạo một Column widget như bên dưới:
Row(
children: [
Container(
color: Colors.orange,
child: FlutterLogo(
size: 60.0,
),
),
Container(
color: Colors.blue,
child: FlutterLogo(
size: 60.0,
),
),
Container(
color: Colors.purple,
child: FlutterLogo(
size: 60.0,
),
),
],
)
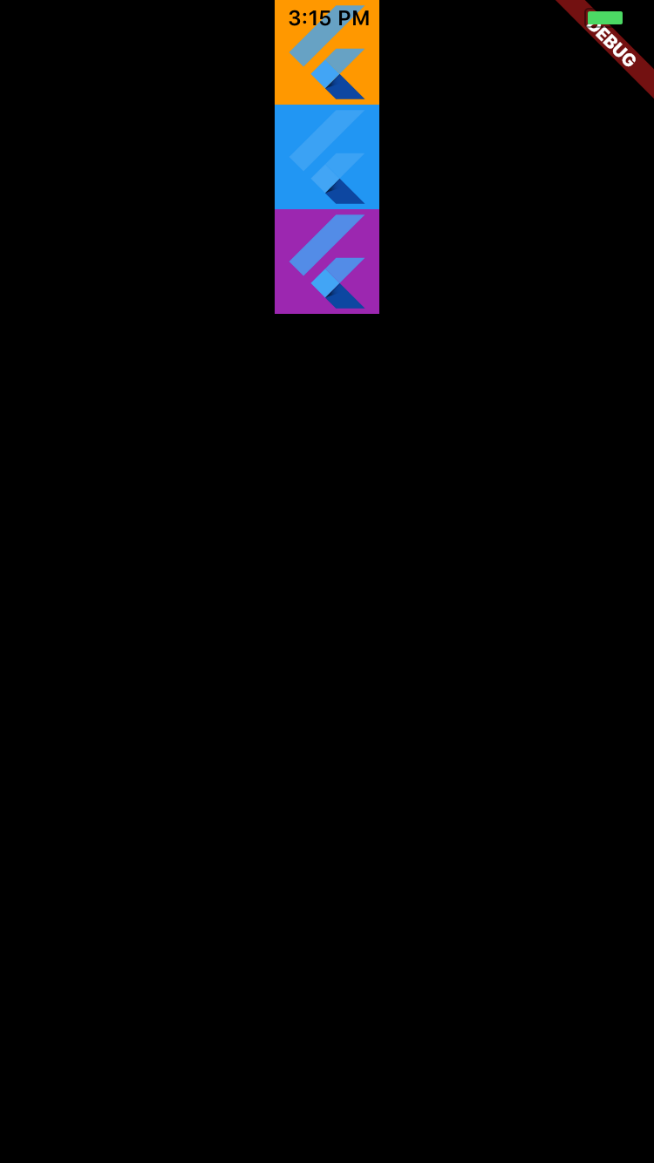
Column và Row có cùng thuộc tính. Vì vậy, trong các ví dụ dưới đây, chúng ta đang làm việc cùng lúc với cả hai widget.
CrossAxis trong Column và Row là gì?
Column(
children: [
Container(
color: Colors.orange,
child: FlutterLogo(
size: 60.0,
),
),
Container(
color: Colors.blue,
child: FlutterLogo(
size: 60.0,
),
),
Container(
color: Colors.purple,
child: FlutterLogo(
size: 60.0,
),
),
],
)
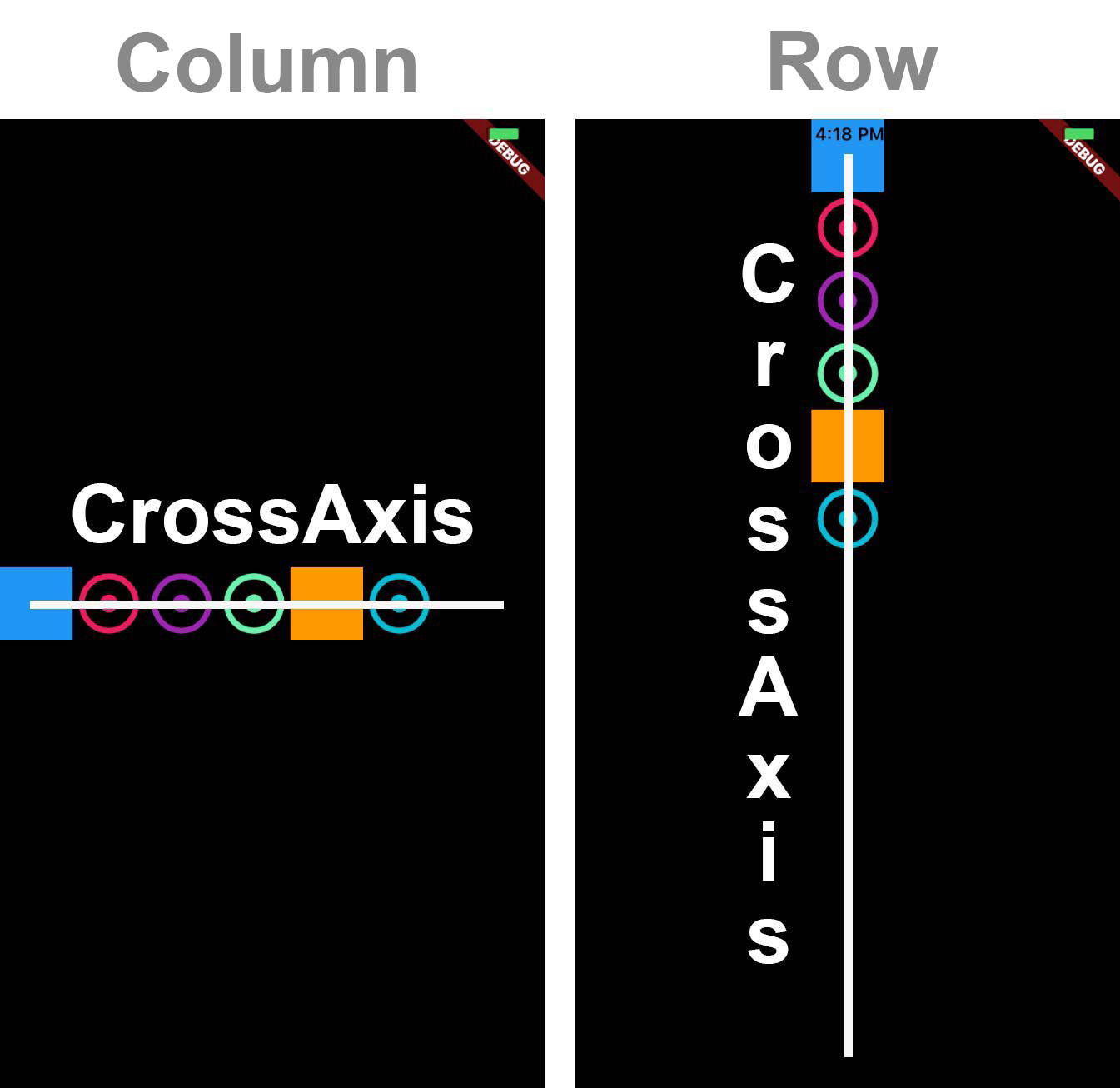
3. CrossAxisAlignment Propery
Chúng ta có thể sử dụng crossAxisAlignment property để căn chỉnh child widget theo hướng mong muốn, ví dụ: crossAxisAlignment.start sẽ đặt các children với cạnh bắt đầu của chúng được căn chỉnh với cạnh bắt đầu của trục chéo.
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
);
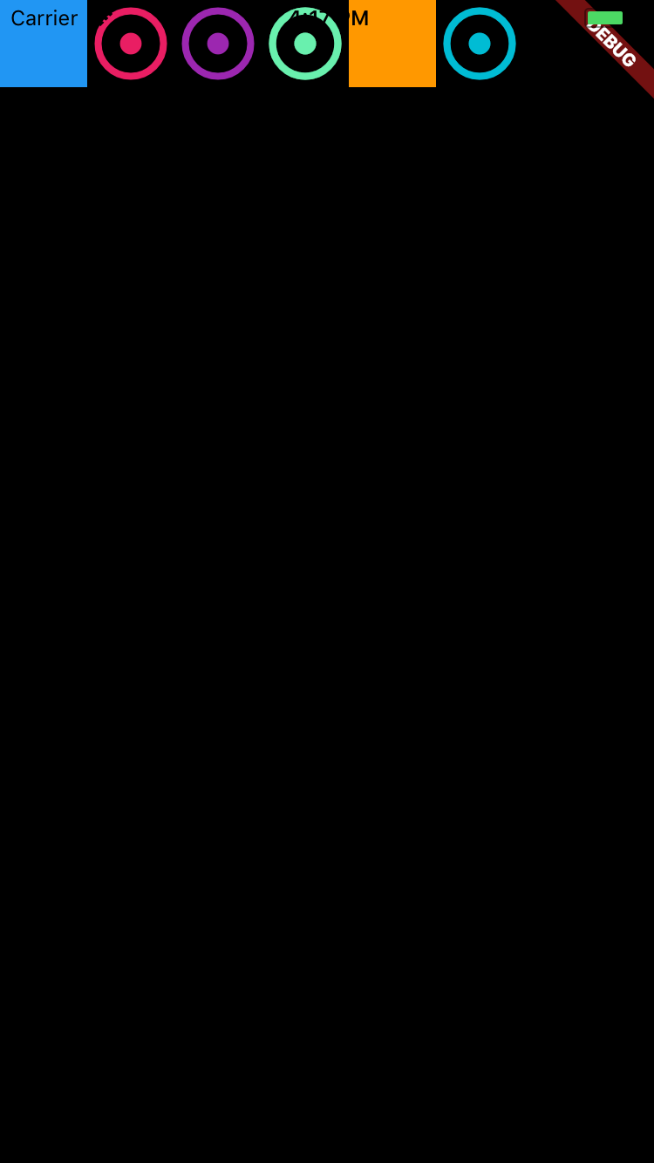
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
);
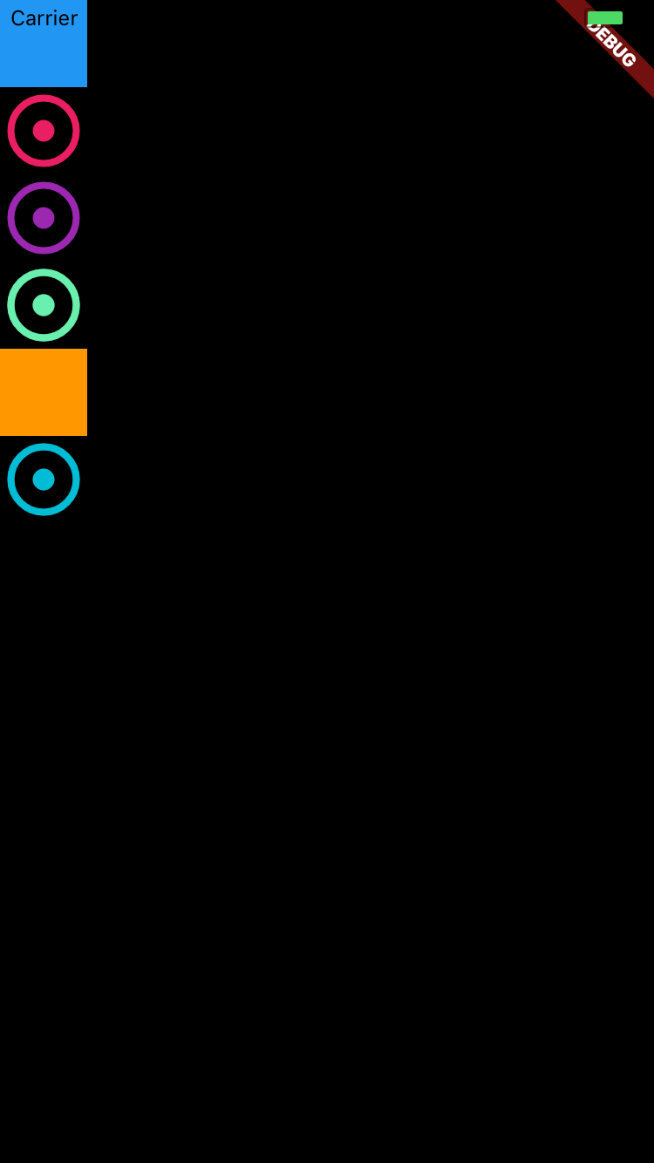
CrossAxisAlignment.center
Đặt các children sao cho tâm của chúng thẳng hàng với giữa trục chéo (cross axis).
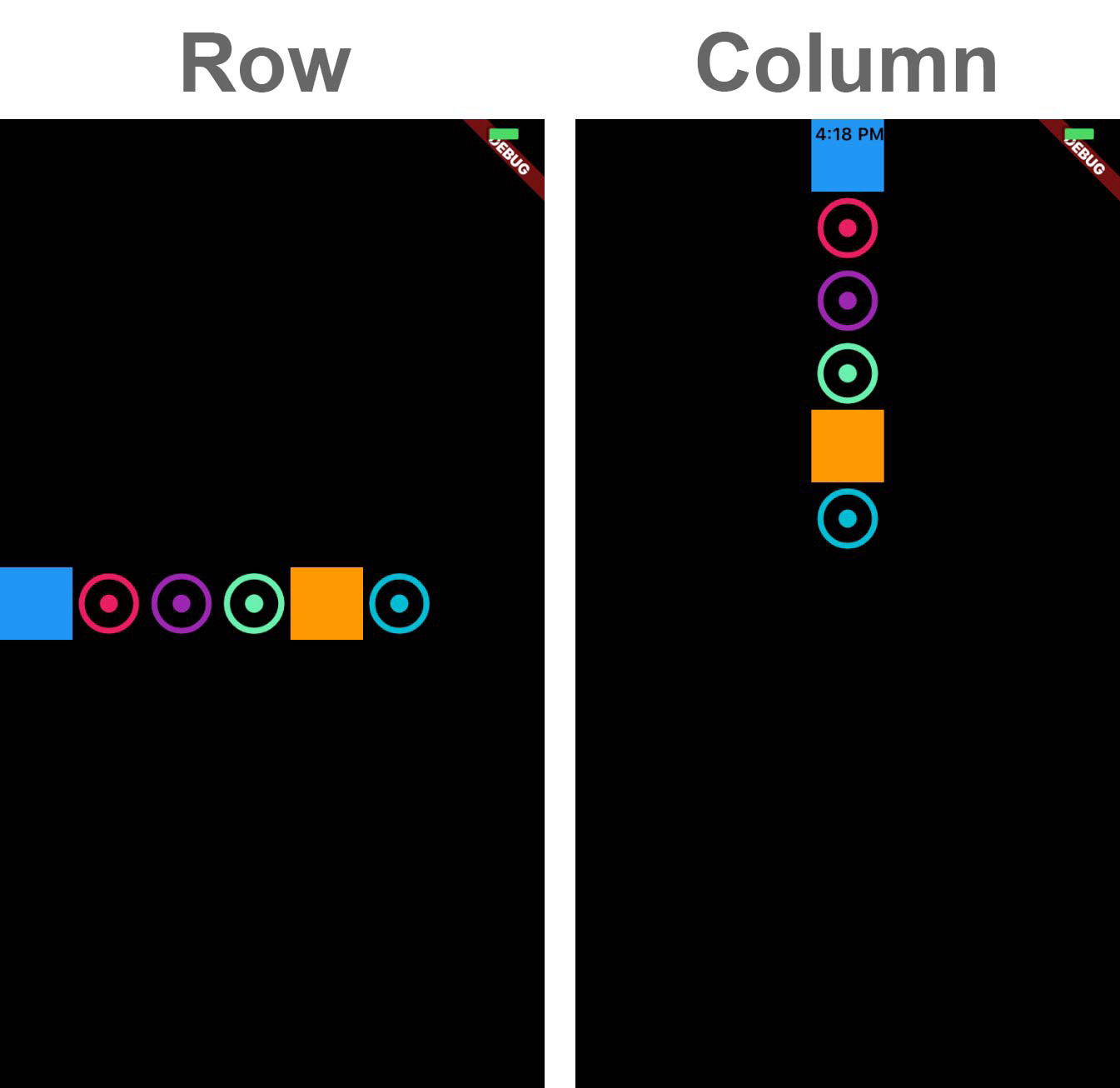
CrossAxisAlignment.end
Đặt các children càng gần cuối trục chéo (cross axis) càng tốt.
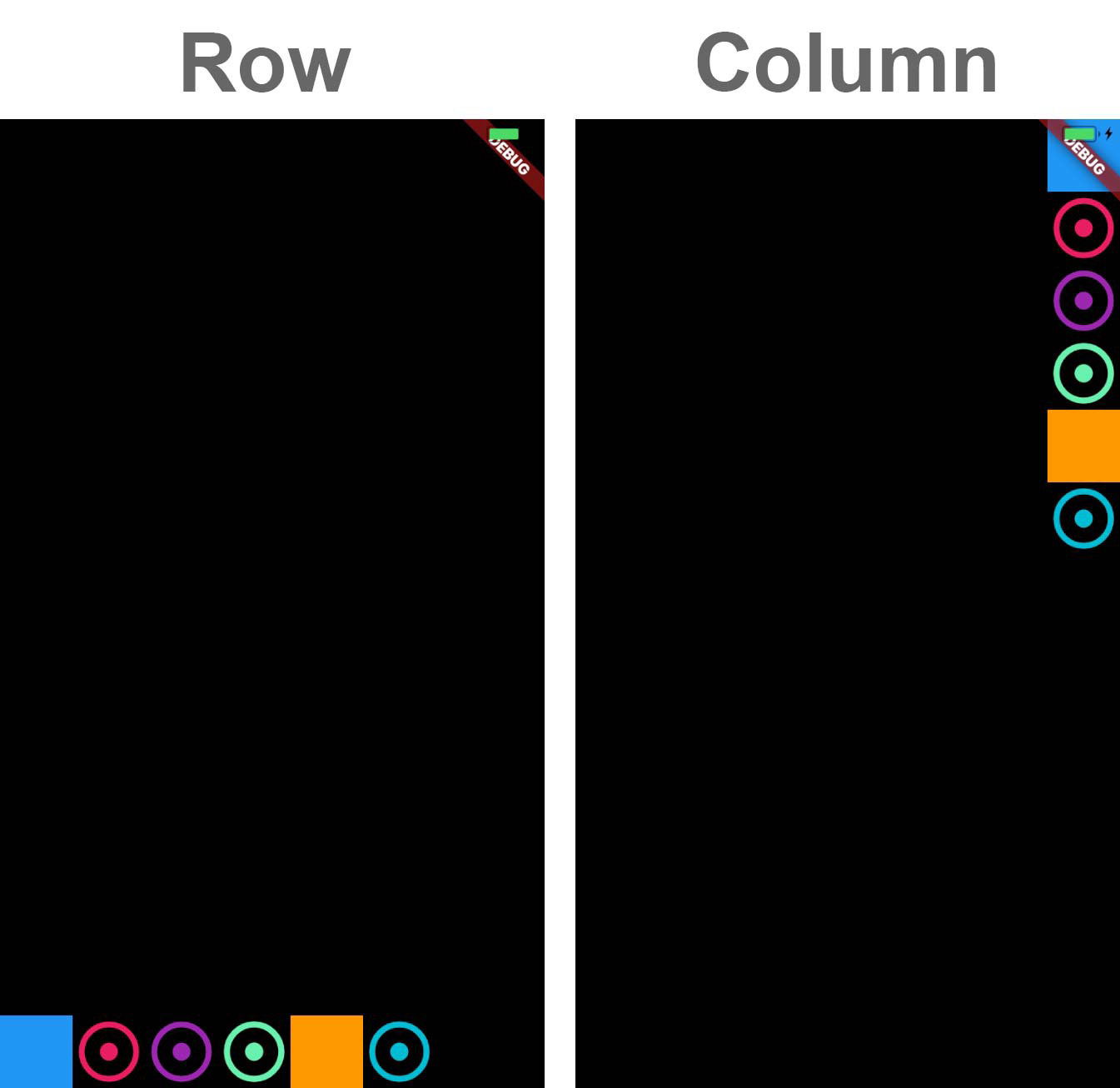
CrossAxisAlignment.stretch
Yêu cầu children để fill ra full chiều dọc hoặc chiều ngang.
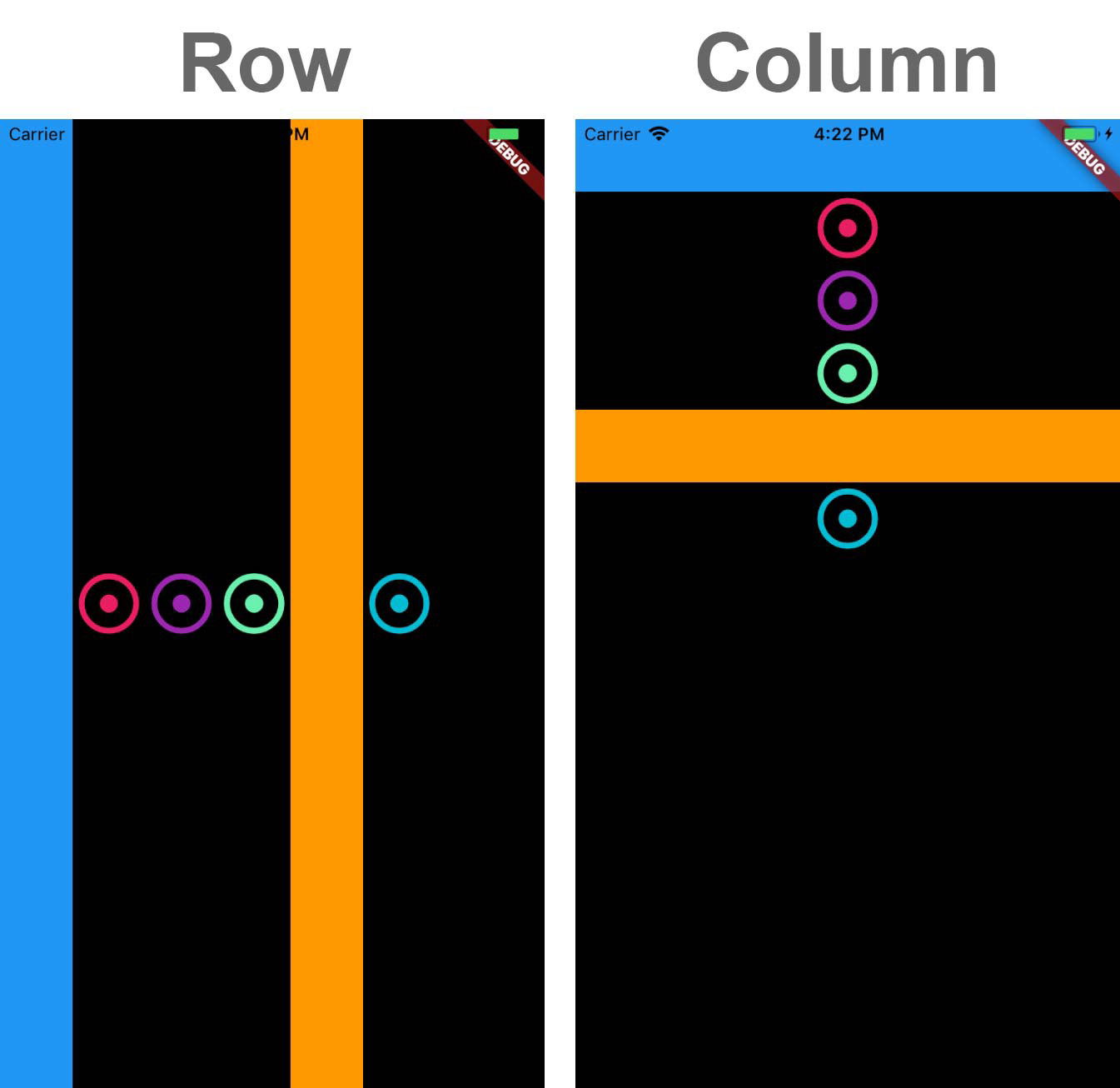
CrossAxisAlignment.baseline
Đặt các children dọc theo trục chéo sao cho các baseline của chúng khớp với nhau.
Bạn nên sử dụng TextBaseline Class với CrossAxisAlignment.baseline.
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
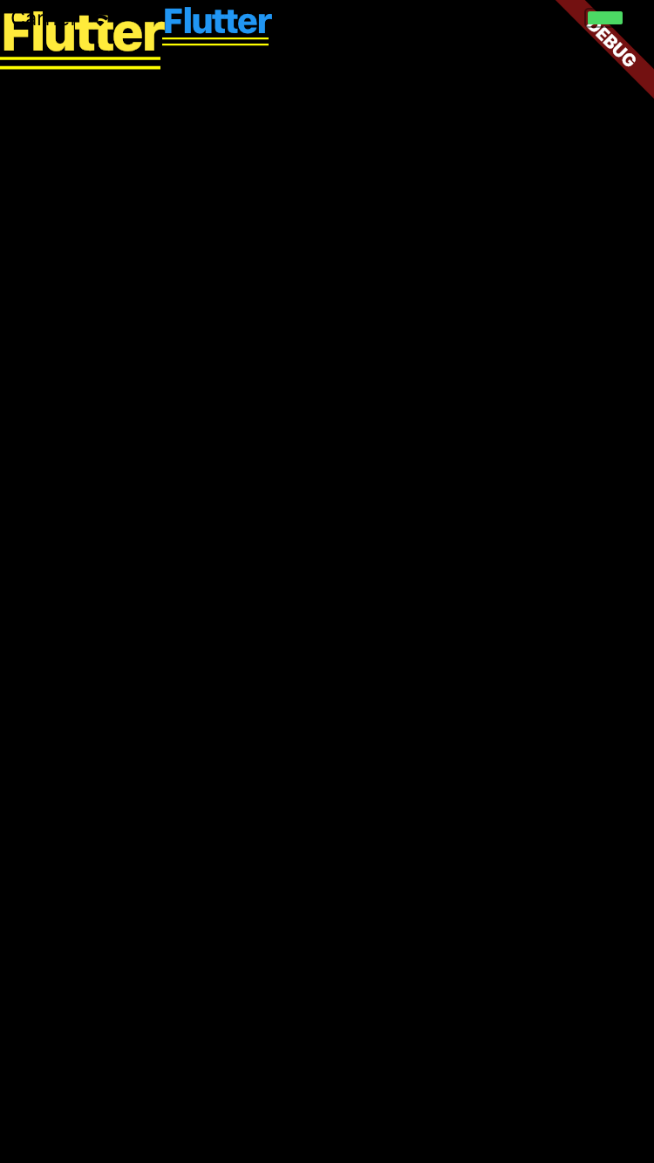
Row(
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: TextBaseline.alphabetic,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
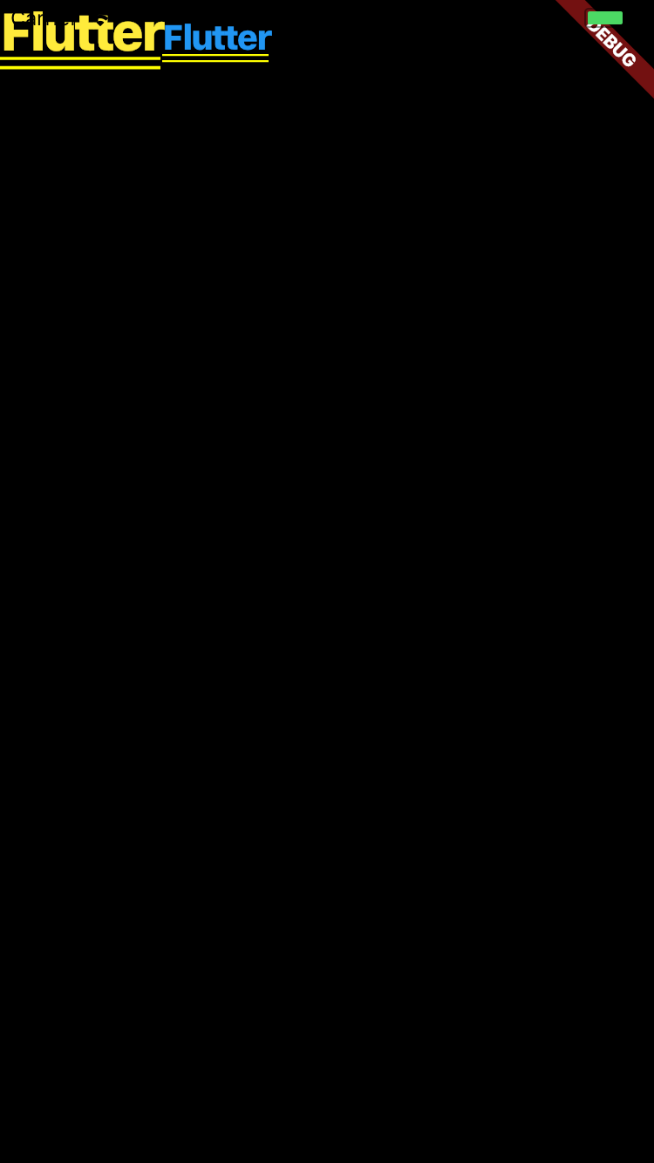
4. TextDirection Propery
Xác định thứ tự sắp xếp children theo chiều ngang và cách diễn giảistart
vàend
theo chiều ngang.
Row(
crossAxisAlignment: CrossAxisAlignment.center,
textDirection: TextDirection.rtl,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
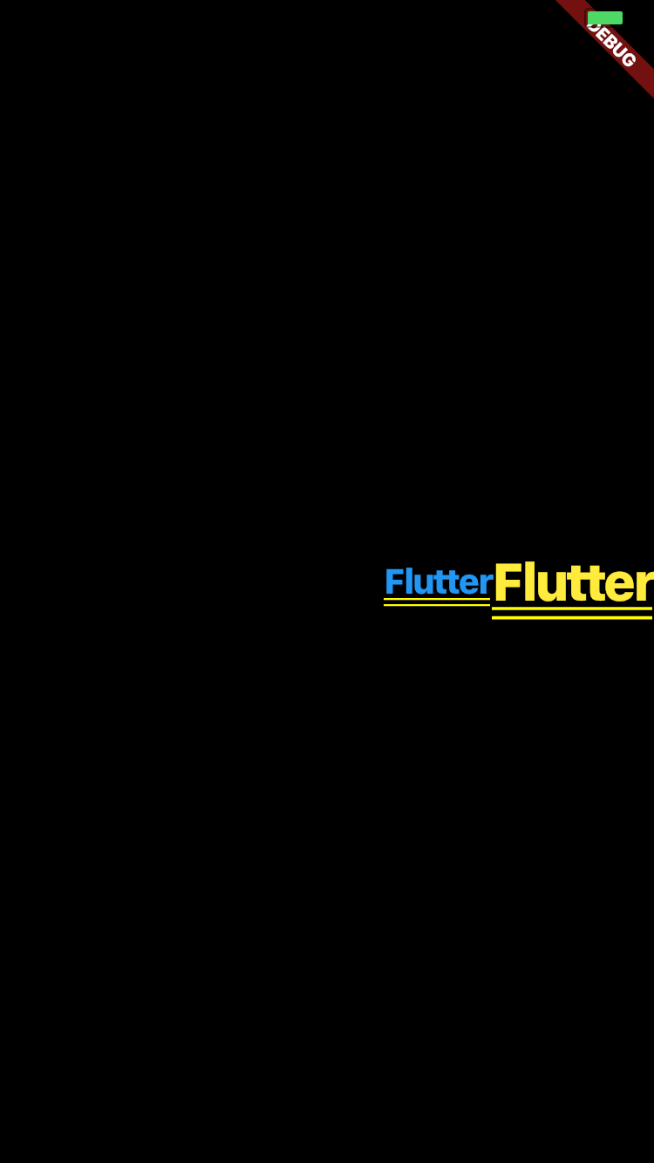
Row(
crossAxisAlignment: CrossAxisAlignment.center,
textDirection: TextDirection.ltr,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
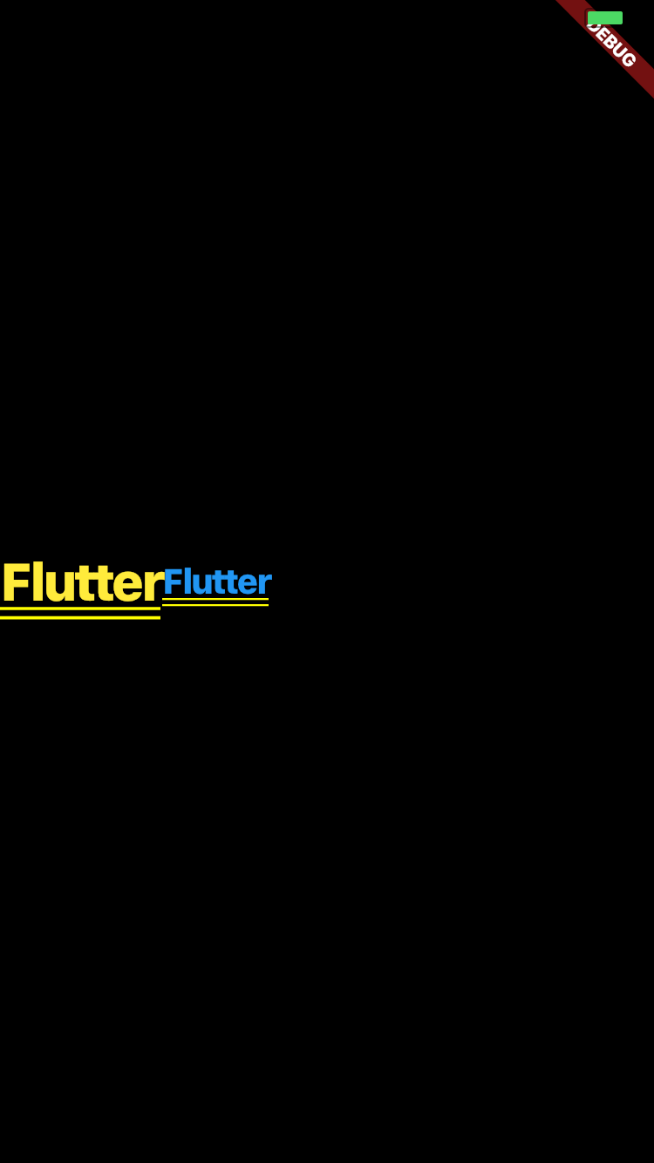
Column(
crossAxisAlignment: CrossAxisAlignment.start,
textDirection: TextDirection.ltr,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
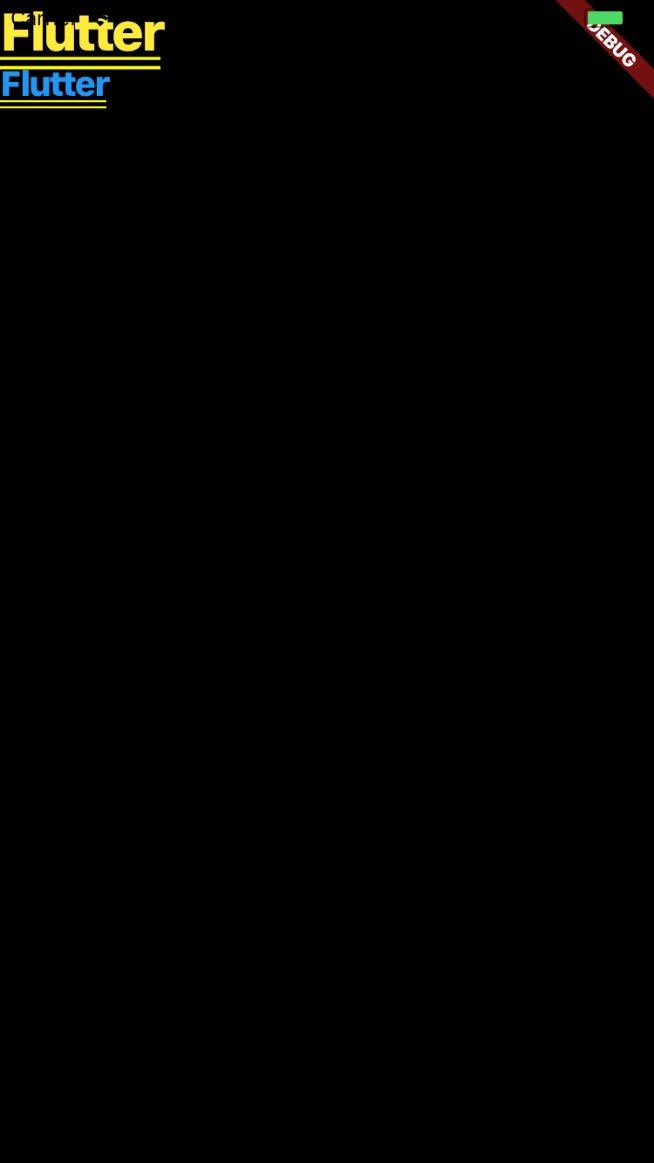
Column(
crossAxisAlignment: CrossAxisAlignment.start,
textDirection: TextDirection.rtl,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
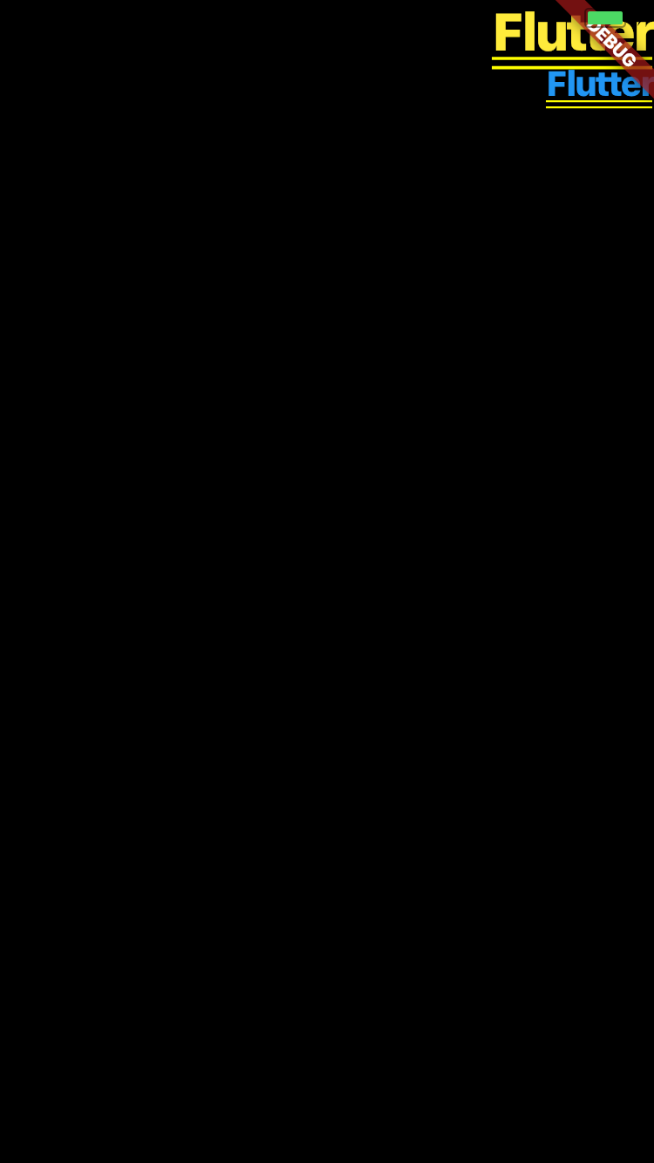
5. VerticalDirection Propery
Xác định thứ tự sắp xếp children theo chiều dọc và cách diễn giải start
vàend
theo chiều dọc.
Mặc định là Vertical Direction.down.
Row(
crossAxisAlignment: CrossAxisAlignment.start,
verticalDirection: VerticalDirection.down,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
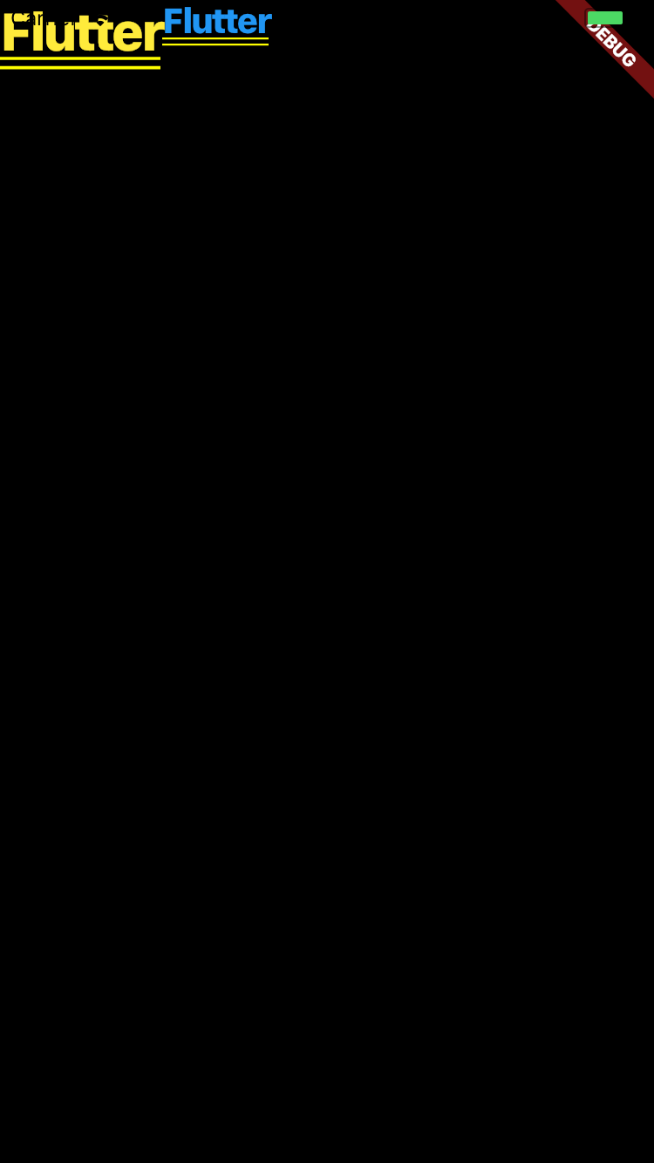
Row(
crossAxisAlignment: CrossAxisAlignment.start,
verticalDirection: VerticalDirection.up,
children: [
Text(
'Flutter',
style: TextStyle(
color: Colors.yellow,
fontSize: 30.0
),
),
Text(
'Flutter',
style: TextStyle(
color: Colors.blue,
fontSize: 20.0
),
),
],
);
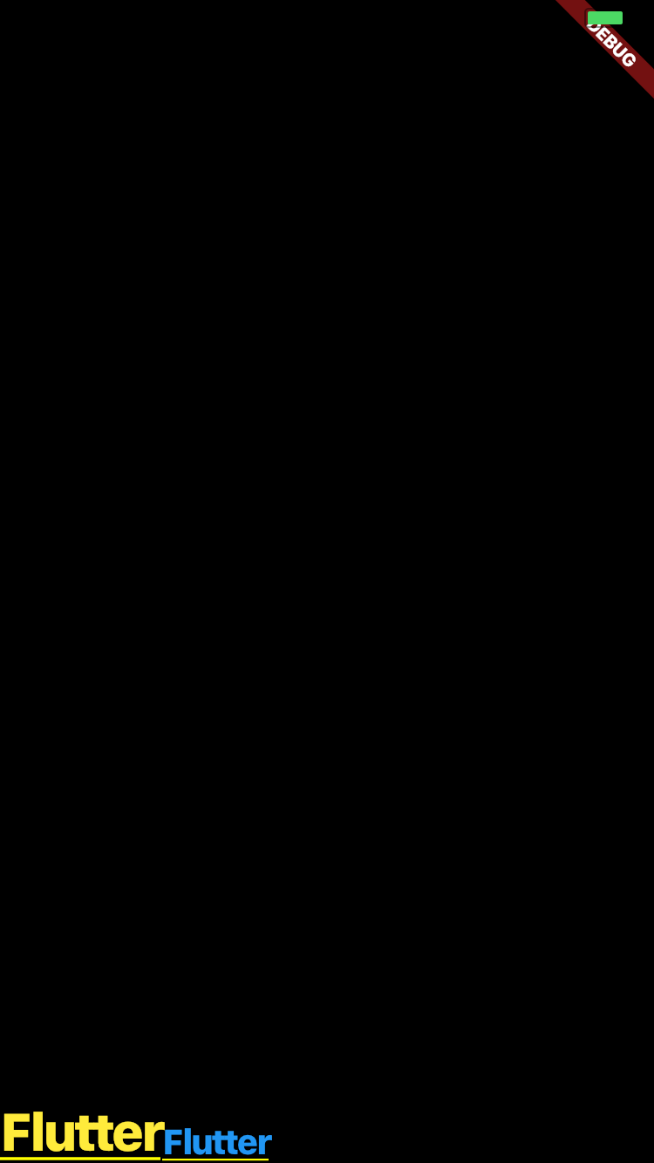
MainAxis trong Row và Column là gì?
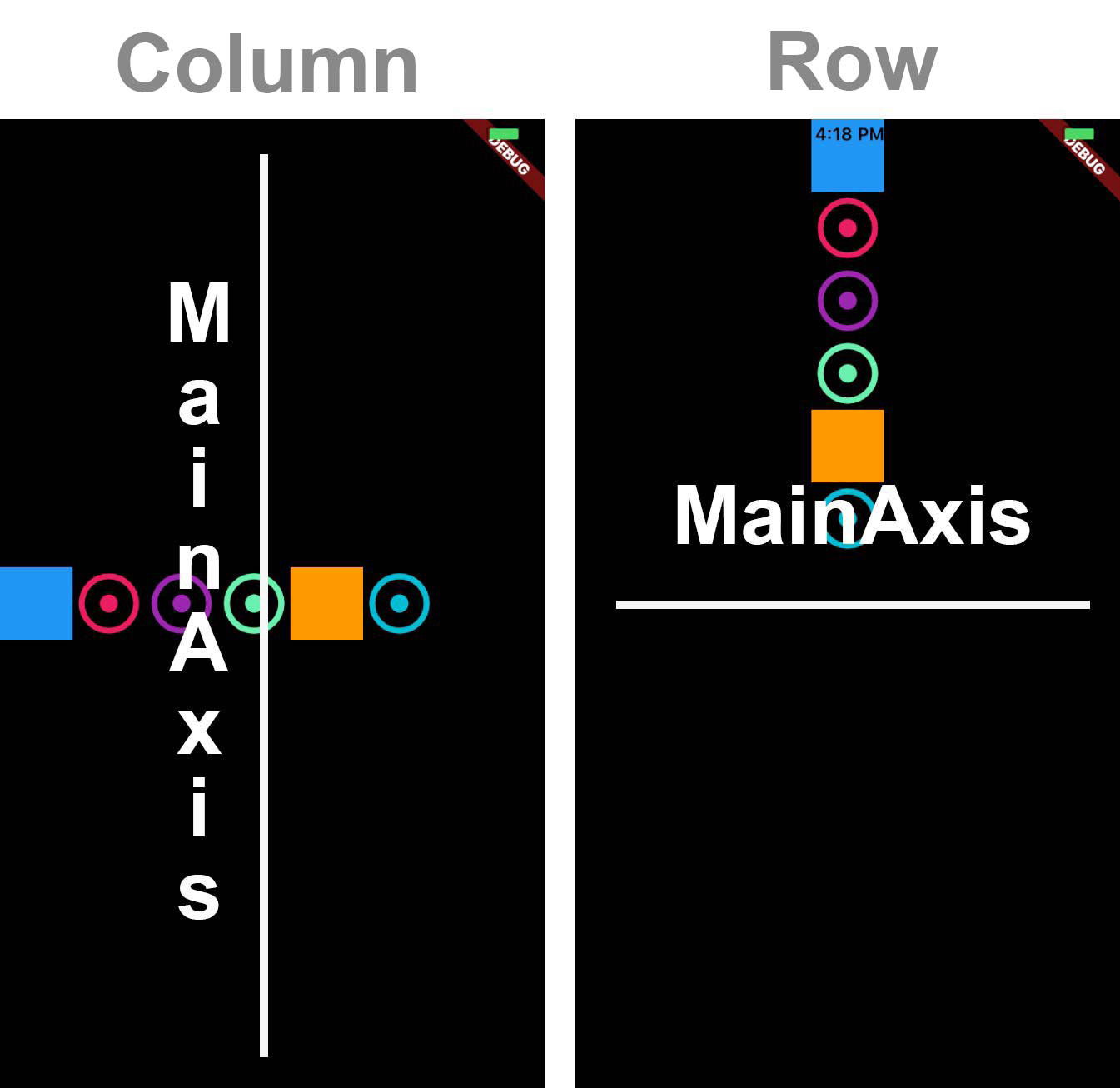
6. MainAxisAlignment Propery
Vị trí của các child widget trên trục chính.
MainAxisAlignment.start
Đặt các children càng gần đầu trục chính càng tốt.
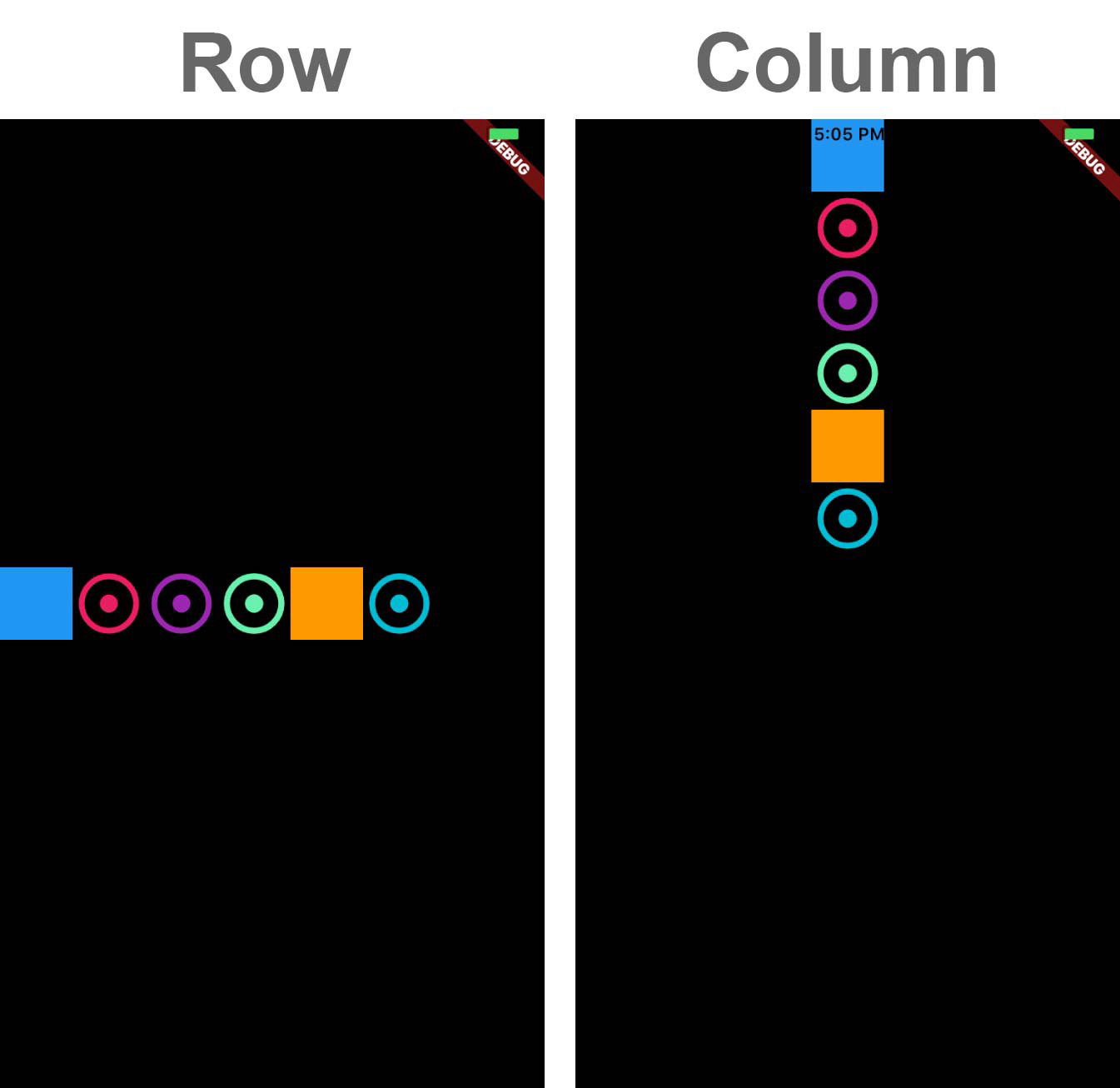
MainAxisAlignment.center
Đặt các children càng gần giữa trục chính càng tốt.
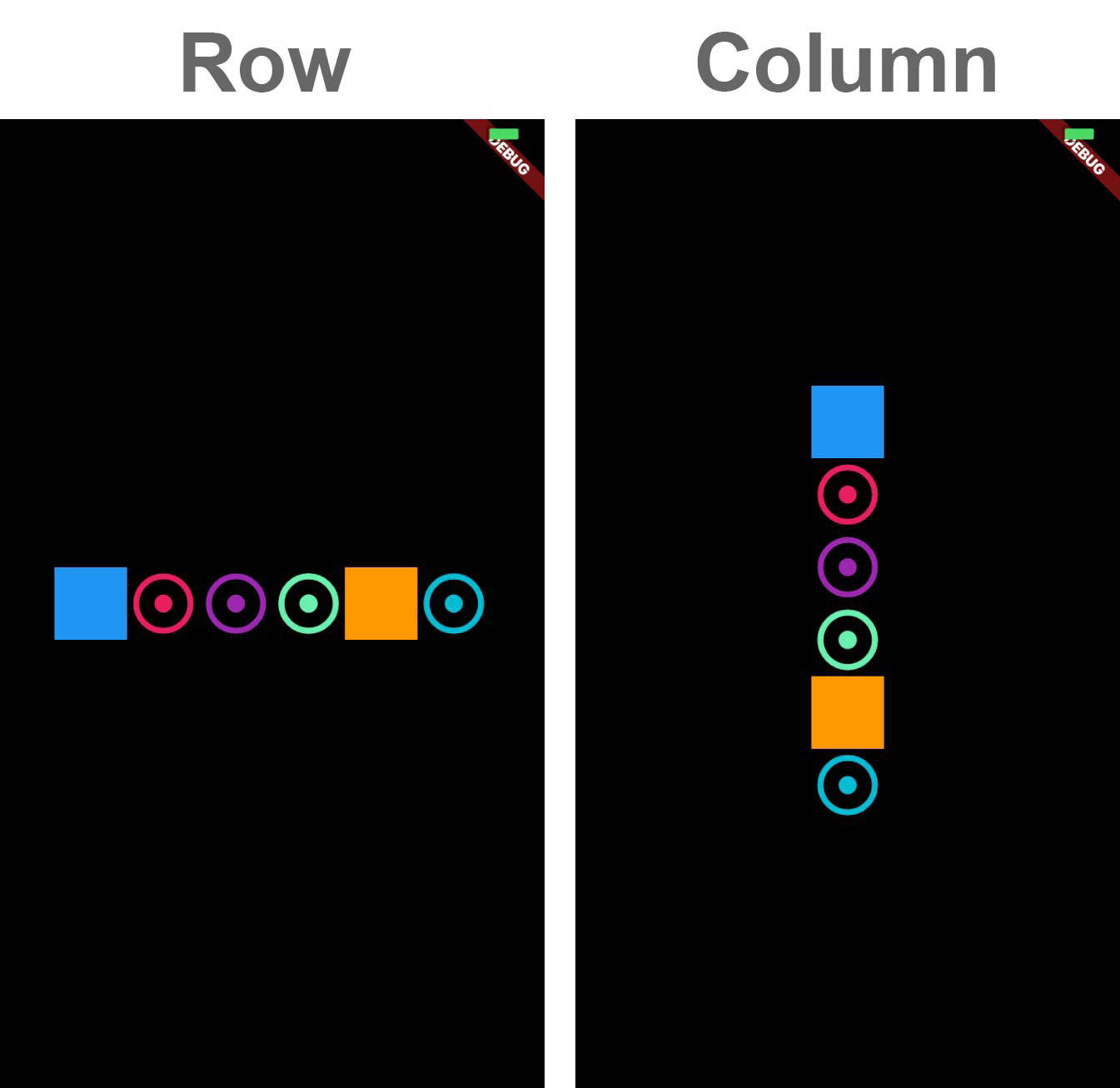
MainAxisAlignment.end
Đặt các children càng gần cuối trục chính càng tốt.
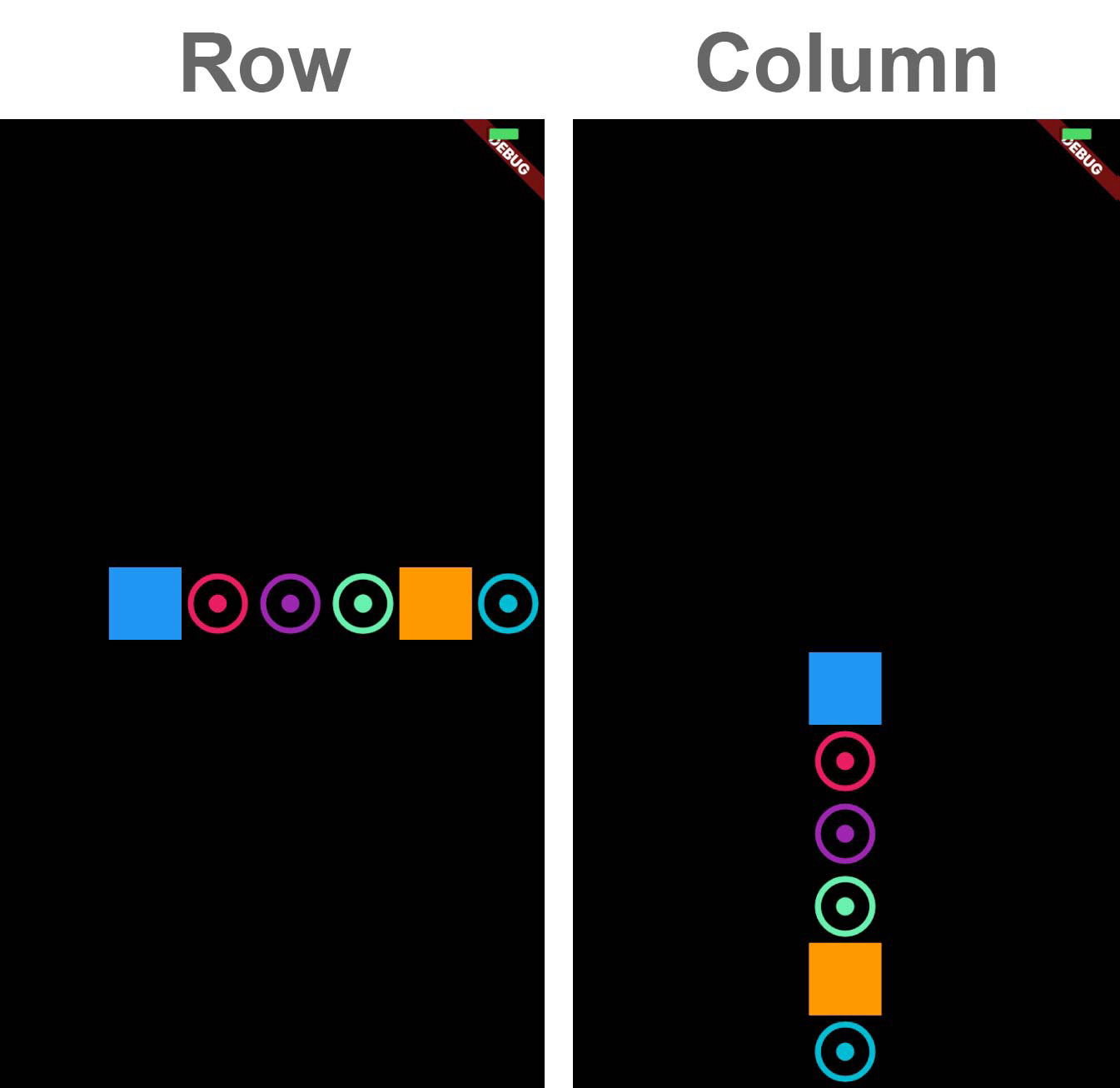
MainAxisAlignment.spaceAround
Đặt đều không gian trống giữa các children cũng như một nửa không gian đó trước và sau children đầu tiên và children cuối cùng.
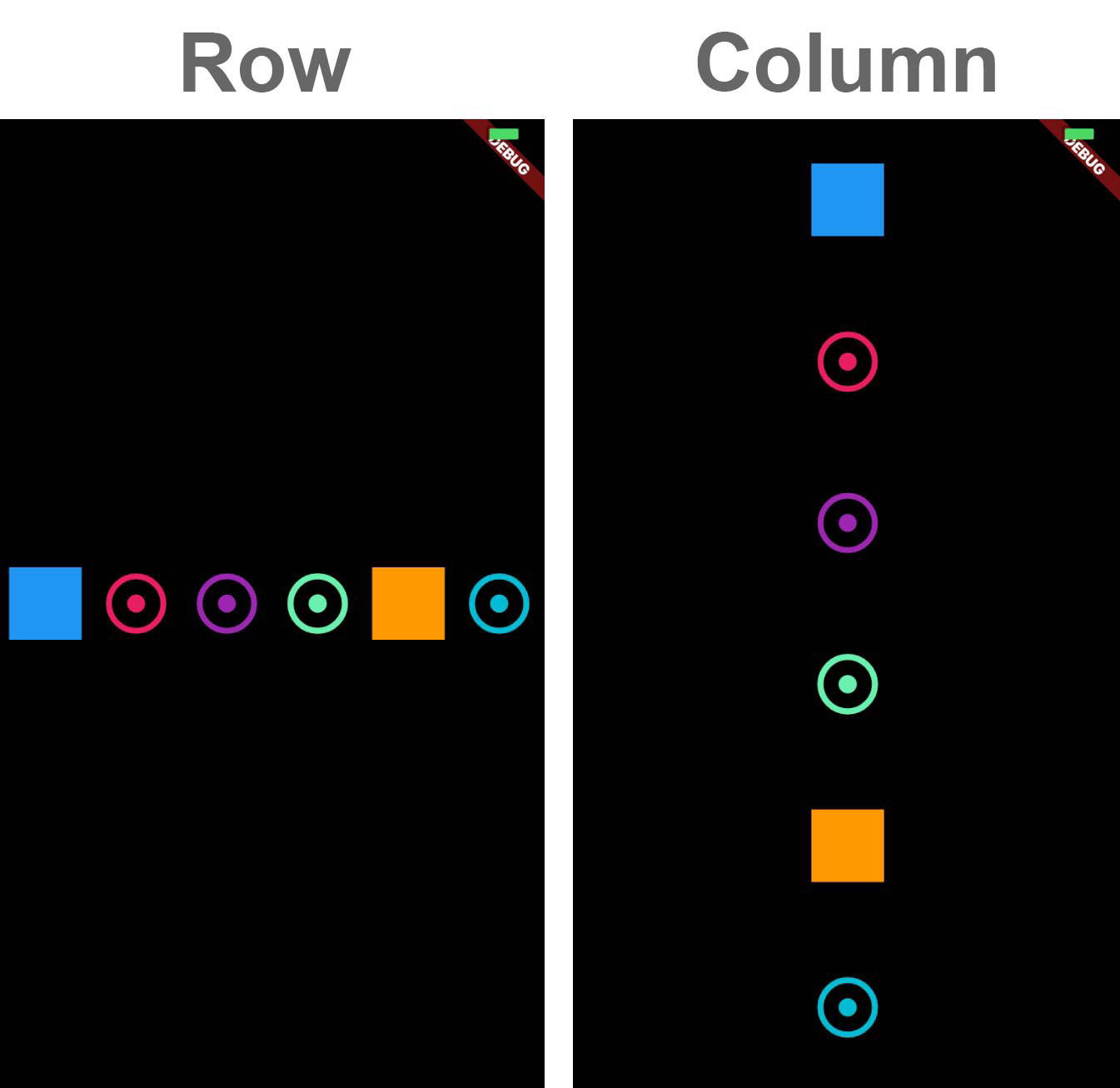
MainAxisAlignment.spaceBetween
Đặt không gian trống giữa các children cách đều nhau.
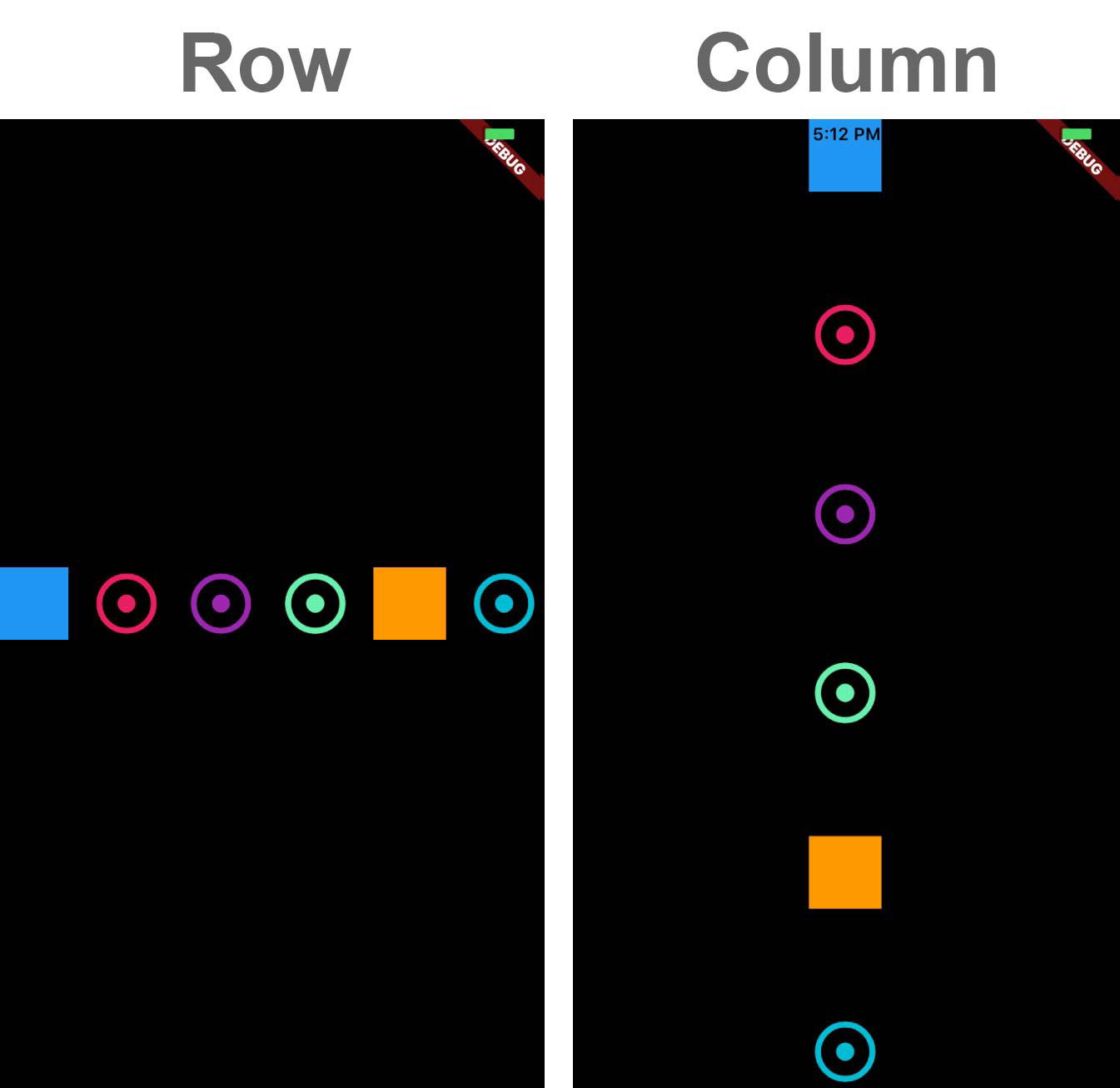
MainAxisAlignment.spaceEvenly
Đặt không gian trống đồng đều giữa các children cũng như trước và sau children đầu tiên và children cuối cùng.
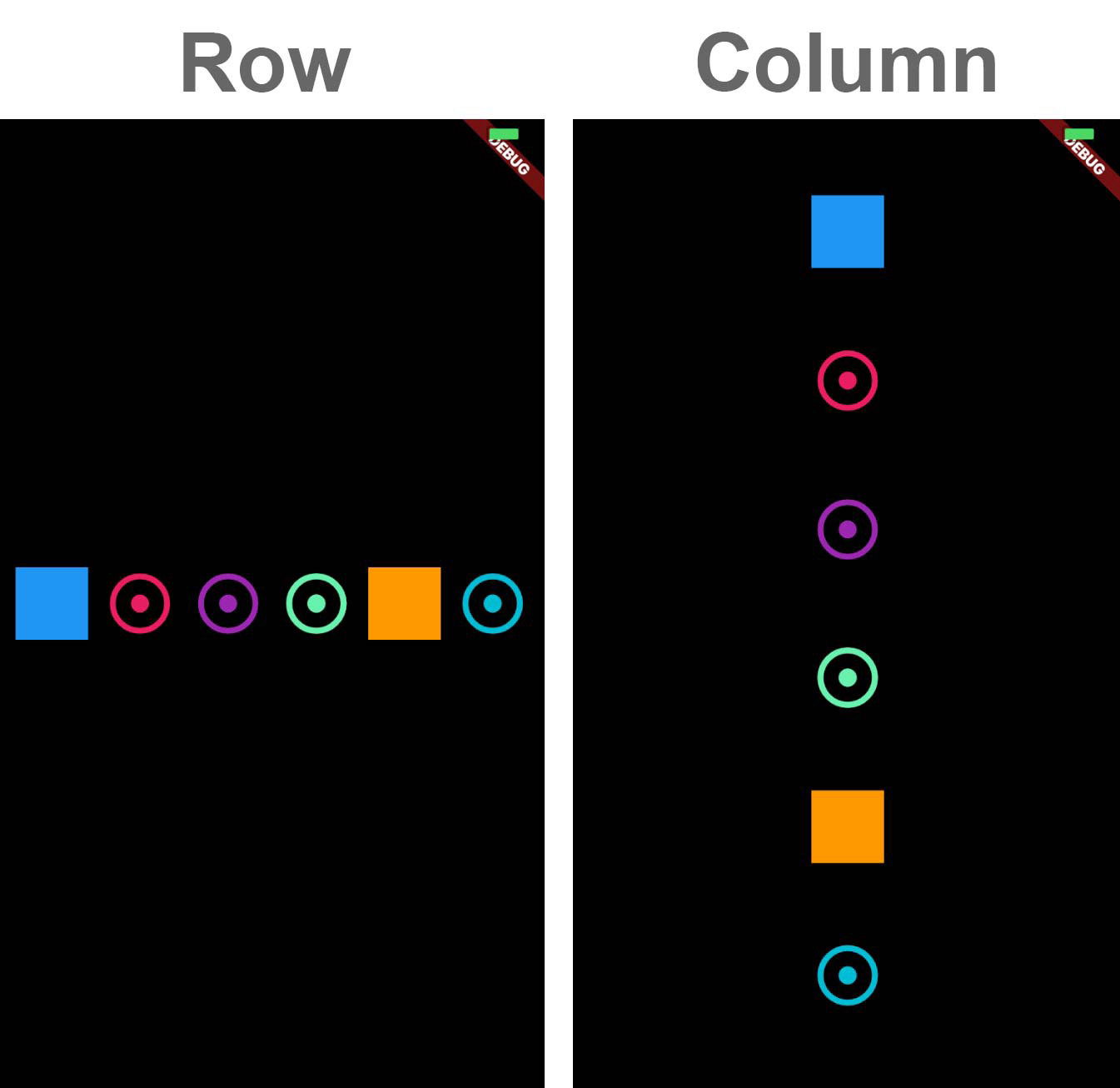
7. MainAxisSize Propery
Kích thước sẽ được phân bổ cho widget trên trục chính.
MainAxisSize.max
Tối đa hóa lượng không gian trống dọc theo trục chính, tùy thuộc vào các hạn chế về incoming layout.
Center(
child: Container(
color: Colors.yellow,
child: Row(
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
),
),
);
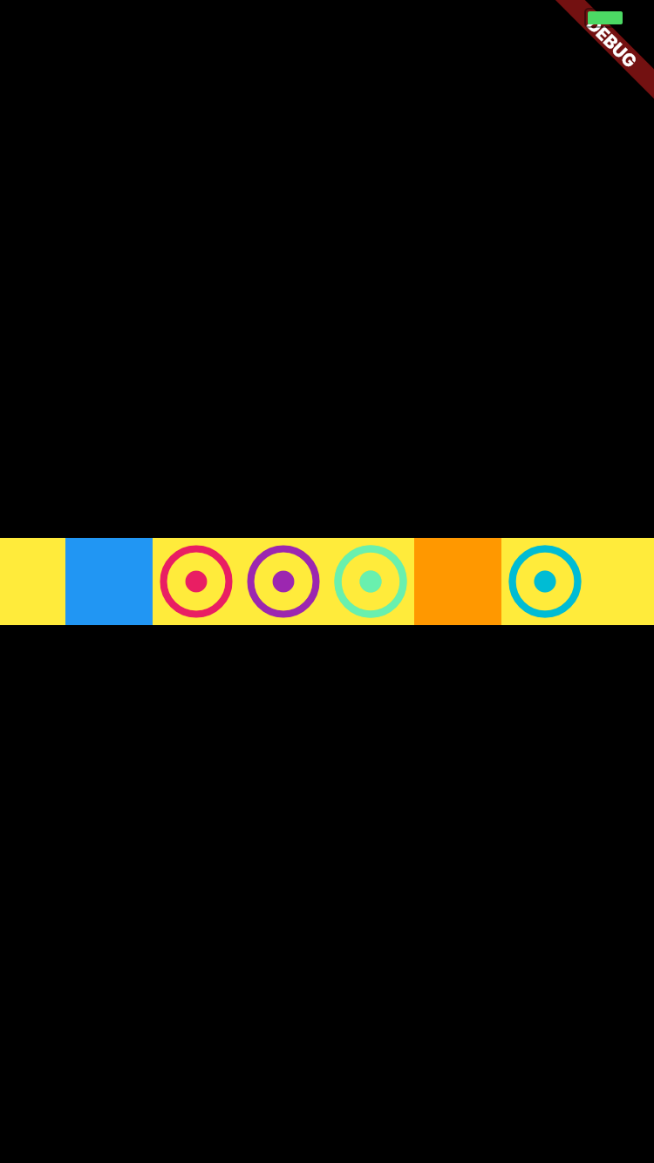
Center(
child: Container(
color: Colors.yellow,
child: Column(
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
),
),
);
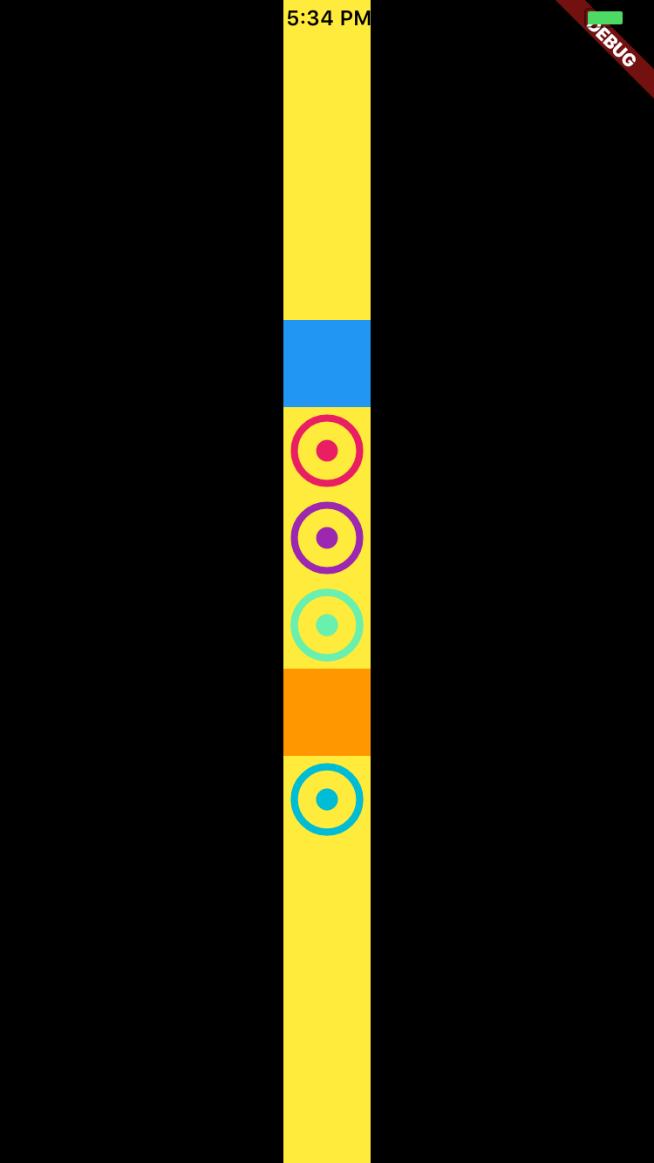
MainAxisSize.min
Giảm thiểu dung lượng trống dọc theo trục chính, tùy thuộc vào các ràng buộc về incoming layout.
Center(
child: Container(
color: Colors.yellow,
child: Row(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
),
),
);
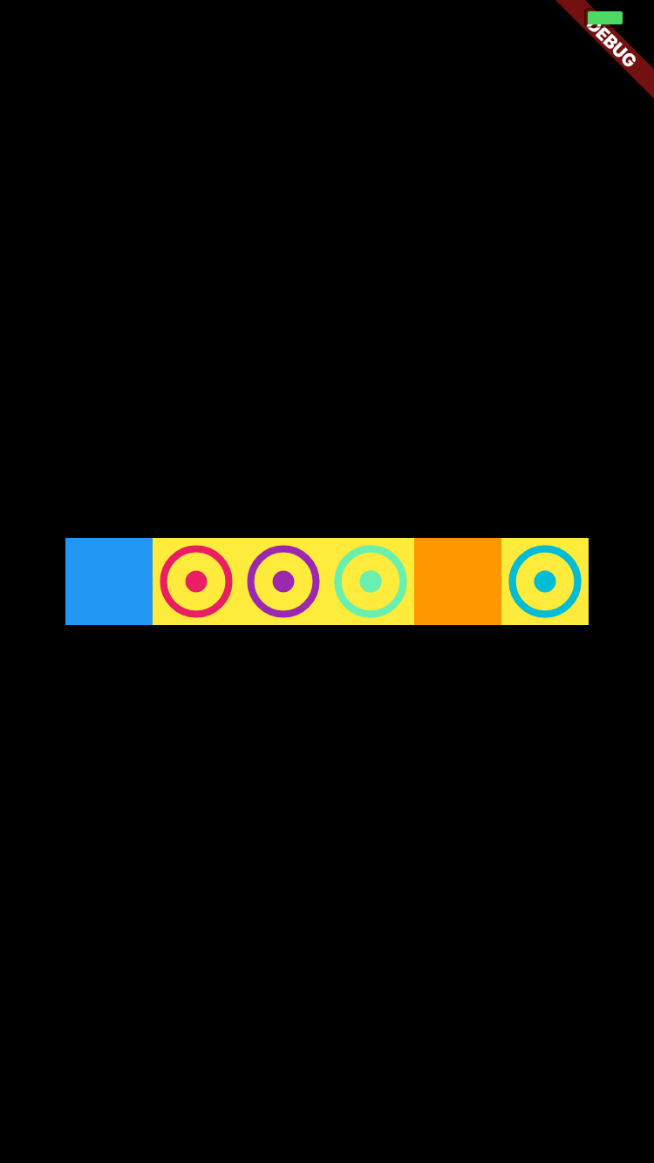
Center(
child: Container(
color: Colors.yellow,
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
color: Colors.blue,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.pink),
Icon(Icons.adjust, size: 50.0, color: Colors.purple,),
Icon(Icons.adjust, size: 50.0, color: Colors.greenAccent,),
Container(
color: Colors.orange,
height: 50.0,
width: 50.0,
),
Icon(Icons.adjust, size: 50.0, color: Colors.cyan,),
],
),
),
);
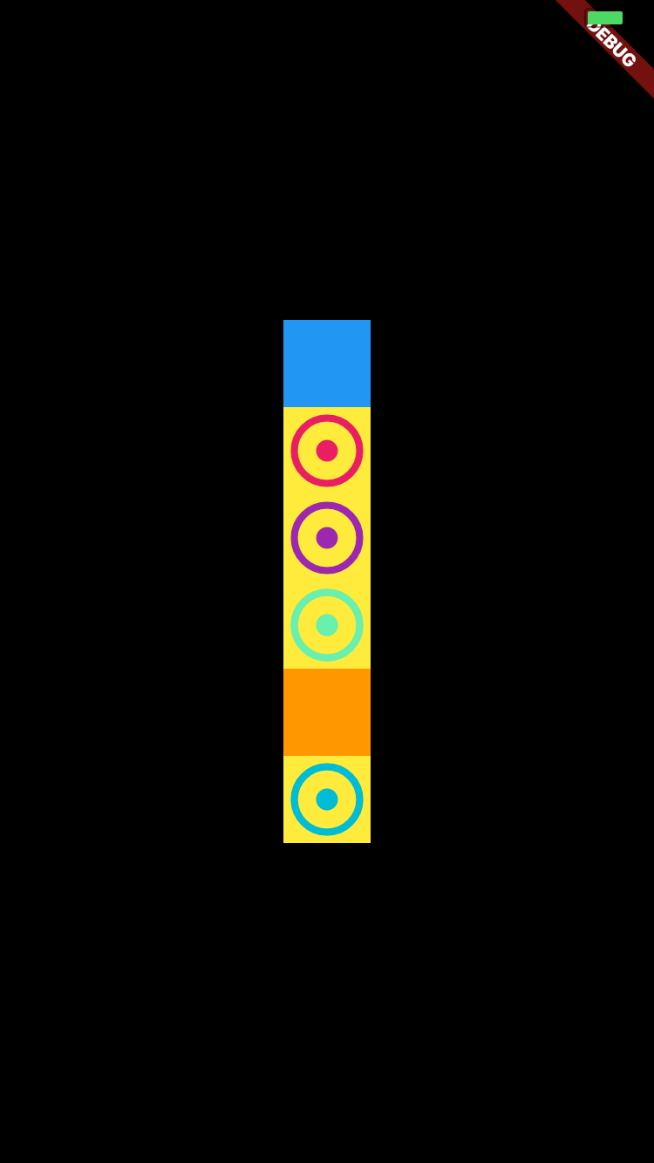
Hi vọng bạn thích bài viết này.
Bài viết được lược dịch từ Julien Louage.
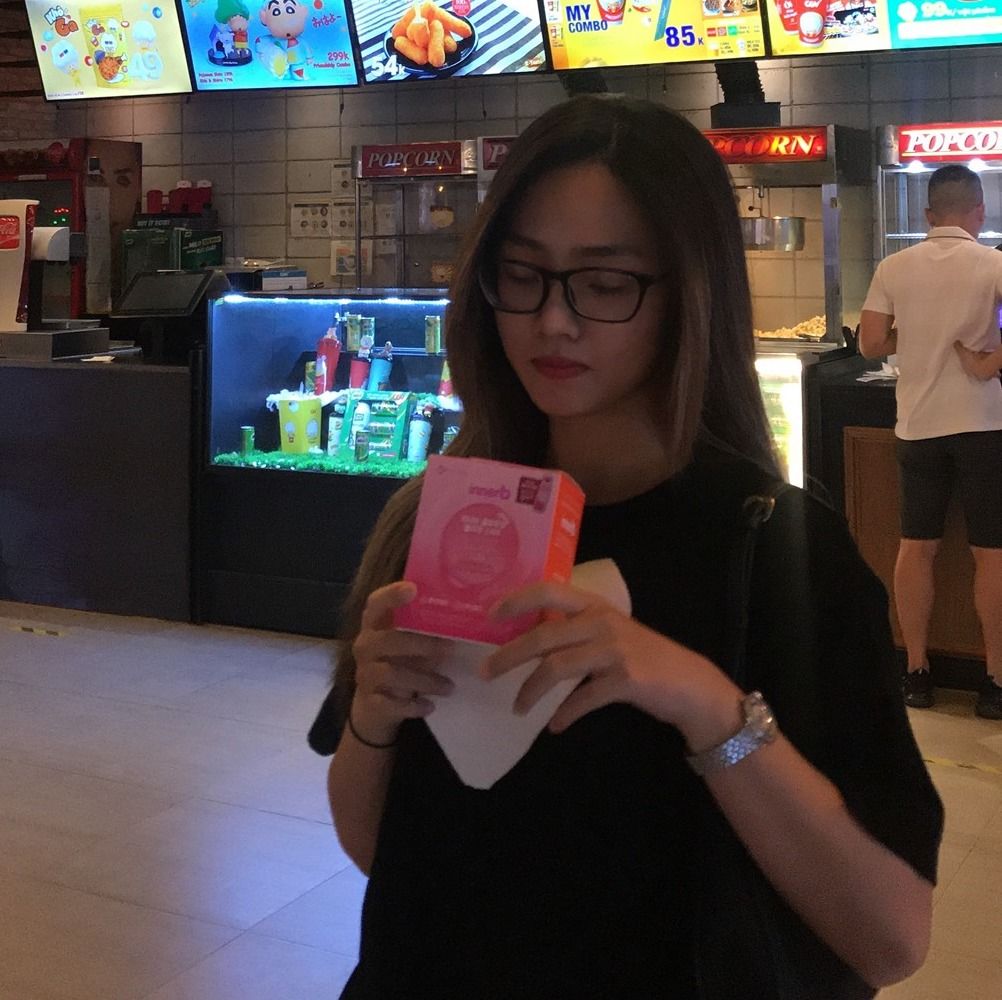
Chau Le
Cực thích đồ ngọt như sữa, kem. Thích phiêu lưu mạo hiểm nhưng vì Covid mà vô tình trở thành người chia sẻ nội dung lập trình :))
follow me :
Bài viết liên quan
Tự học Dart: Các Dart Operators (toán tử) bạn cần biết
Sep 02, 2023 • 18 min read
Flutter cơ bản: Điều cần biết khi lập trình ứng dụng đầu tiên
Aug 23, 2023 • 13 min read
Dart là gì? Giới thiệu cơ bản về ngôn ngữ lập trình Dart
Aug 21, 2023 • 11 min read
Flutter là gì? Vì sao nên học công cụ lập trình Flutter?
Aug 19, 2023 • 11 min read
Flutter cơ bản: Widget Tree, Element Tree & Render Tree
Aug 19, 2023 • 10 min read
So sánh StatelessWidget và StatefulWidget
Jul 17, 2022 • 12 min read