Chúng ta biết việc navigate từ 1 route này sang bất kỳ route khác trong Flutter dễ dàng như thế nào. Chúng ta chỉ cần push và pop.
Để push sang màn hình khác:
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondRoute()),
);
Để pop màn hình hiện tại:
Navigator.pop(context);
Đơn giản vậy thôi. NHƯNG… Nó rất nhàm chán, không có animation nào cả 😦.
Tại Winkl khi chúng ta bắt đầu play với các animation, chúng ta nhận ra rằng page transition thực sự có thể làm cho giao diện người dùng của bạn đẹp hơn. Nếu bạn muốn có một slide transition giống như iOS, bạn sử dụng CupertinoPageRoute. Chỉ vậy, không có gì khác.
Navigator.push(
context, CupertinoPageRoute(builder: (context) => Screen2()))
Nhưng đối với việc custom transition, Flutter cung cấp cho chúng ta các transition widget khác nhau. Hãy xem cách chúng ta có thể sử dụng chúng.
Chúng ta biết rằng Navigator.push nhận hai tham số (BuildContext context, Route<T> route). Chúng ta có thể tạo page route tùy chỉnh của riêng mình với một số transition animation.. Hãy bắt đầu với một cái gì đó đơn giản như slide transition.
Slide Transition
Chúng ta sẽ mở rộng PageRouteBuilder và định nghĩa transitionsBuilder sẽ trả về SlideTransition widget. SlideTransition widget có vị trí của type Animation<Offset>. Chúng ta sẽ sử dụng Tween<Offset> để cung cấp begin offset và end offset.
import 'package:flutter/material.dart';
class SlideRightRoute extends PageRouteBuilder {
final Widget page;
SlideRightRoute({this.page})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
page,
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
SlideTransition(
position: Tween<Offset>(
begin: const Offset(-1, 0),
end: Offset.zero,
).animate(animation),
child: child,
),
);
}
Bây giờ chúng ta có thể sử dụng SlideRightRoute thay vì MaterialPageRoute như thế này.
Navigator.push(context, SlideRightRoute(page: Screen2()))
Kết quả là…
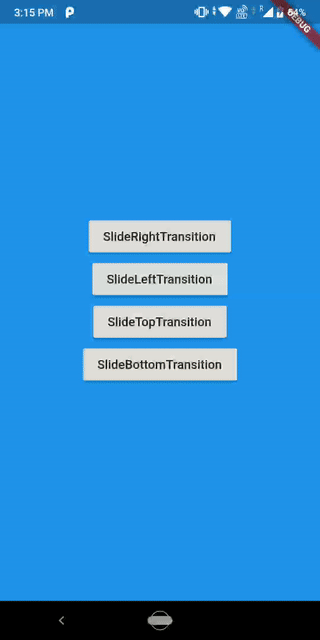
Khá dễ dàng phải không? Bạn có thể thay đổi slide transition bằng cách thay đổi offset.
Scale Transition
Scale Transition làm hoạt ảnh scale của một widget được chuyển đổi (transformed widget). Bạn cũng có thể thay đổi cách hoạt ảnh xuất hiện bằng cách thay đổi các curve của CurvedAnimation. Trong ví dụ dưới đây, mình đã sử dụng Curves.fastOutSlowIn.
Kết quả là...
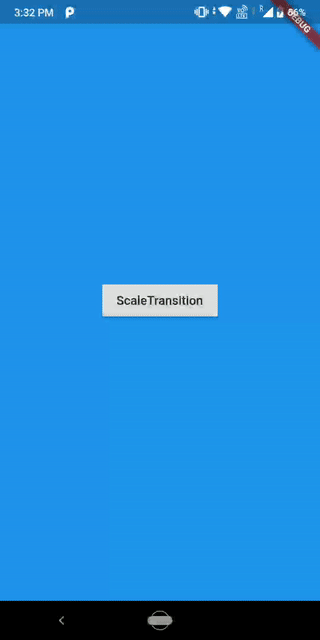
Rotation Transition
Rotation transition làm animate rotation của một widget. Bạn cũng có thể cung cấp transitionDuration cho PageRouteBuilder của mình.
import 'package:flutter/material.dart';
class RotationRoute extends PageRouteBuilder {
final Widget page;
RotationRoute({this.page})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
page,
transitionDuration: Duration(seconds: 1),
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
RotationTransition(
turns: Tween<double>(
begin: 0.0,
end: 1.0,
).animate(
CurvedAnimation(
parent: animation,
curve: Curves.linear,
),
),
child: child,
),
);
}
Kết quả là…
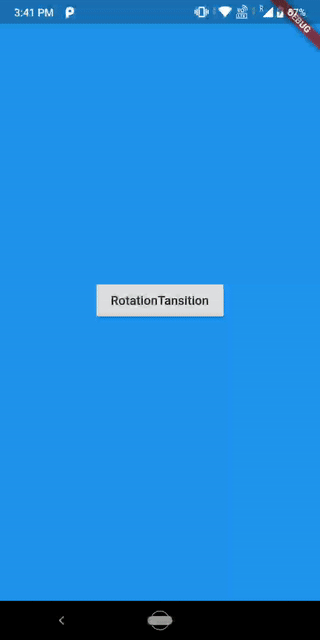
Size Transition
import 'package:flutter/material.dart';
class SizeRoute extends PageRouteBuilder {
final Widget page;
SizeRoute({this.page})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
page,
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
Align(
child: SizeTransition(
sizeFactor: animation,
child: child,
),
),
);
}
Kết quả là…
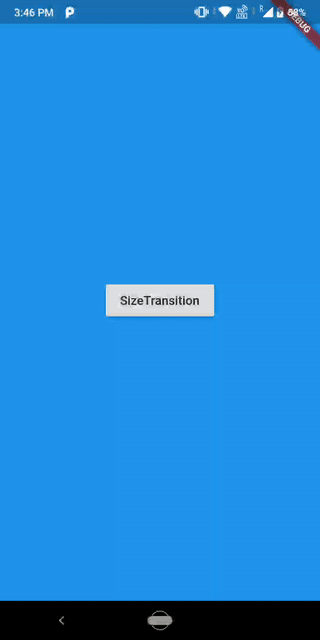
Fade Transition
import 'package:flutter/material.dart';
class FadeRoute extends PageRouteBuilder {
final Widget page;
FadeRoute({this.page})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
page,
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
FadeTransition(
opacity: animation,
child: child,
),
);
}
Kết quả là…
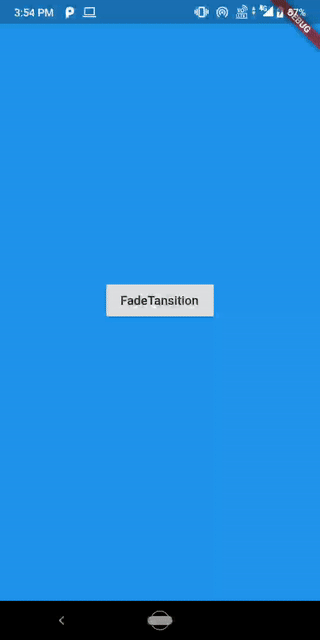
Tuyệt vời!! Chúng ta đã thấy tất cả các transition cơ bản.
Bây giờ chúng ta hãy làm điều gì đó cải tiến hơn. Điều gì sẽ xảy ra nếu chúng ta muốn tạoanimate cho cả hai route. entering route(new page) và exit route(old page). Chúng ta có thể sử dụng các stack transition animations và áp dụng nó cho cả hai route. Một ví dụ về điều này có thể là slide trong route mới và slide out route cũ. Đây là hoạt ảnh chuyển tiếp yêu thích của mình ❤️. Hãy xem chúng ta có thể làm điều đó như thế nào.
import 'package:flutter/material.dart';
class EnterExitRoute extends PageRouteBuilder {
final Widget enterPage;
final Widget exitPage;
EnterExitRoute({this.exitPage, this.enterPage})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
enterPage,
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
Stack(
children: <Widget>[
SlideTransition(
position: new Tween<Offset>(
begin: const Offset(0.0, 0.0),
end: const Offset(-1.0, 0.0),
).animate(animation),
child: exitPage,
),
SlideTransition(
position: new Tween<Offset>(
begin: const Offset(1.0, 0.0),
end: Offset.zero,
).animate(animation),
child: enterPage,
)
],
),
);
}
Và sử dụng nó như thế này.
Navigator.push(context,
EnterExitRoute(exitPage: this, enterPage: Screen2()))
Và kết quả là...
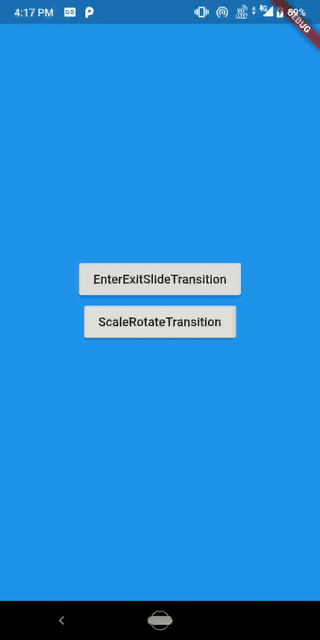
Chúng ta cũng có thể kết hợp nhiều transition để tạo ra thứ gì đó tuyệt vời như scale và rotate cùng một lúc. Đầu tiên, ta có ScaleTransition, child của nó là RotationTransition và child của RotationTransition là page.
import 'package:flutter/material.dart';
class ScaleRotateRoute extends PageRouteBuilder {
final Widget page;
ScaleRotateRoute({this.page})
: super(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) =>
page,
transitionDuration: Duration(seconds: 1),
transitionsBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) =>
ScaleTransition(
scale: Tween<double>(
begin: 0.0,
end: 1.0,
).animate(
CurvedAnimation(
parent: animation,
curve: Curves.fastOutSlowIn,
),
),
child: RotationTransition(
turns: Tween<double>(
begin: 0.0,
end: 1.0,
).animate(
CurvedAnimation(
parent: animation,
curve: Curves.linear,
),
),
child: child,
),
),
);
}
Và kết quả là...
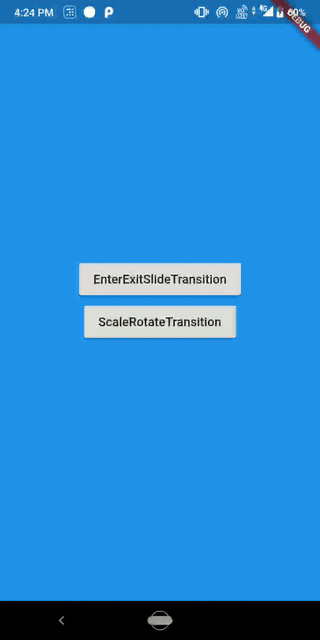
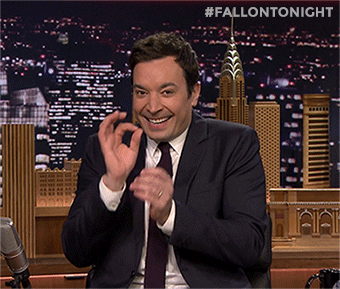
Làm tốt lắm các bạn! Đây là mọi thứ bạn cần biết về route transition animation trong Flutter. Cố gắng kết hợp một số transition và tạo ra một cái gì đó tuyệt vời. Nếu bạn làm được điều gì đó tuyệt vời, đừng quên chia sẻ điều đó với mình. Tất cả source code ở trên GitHub repo.
Bài viết được lược dịch từ Divyanshu Bhargava.
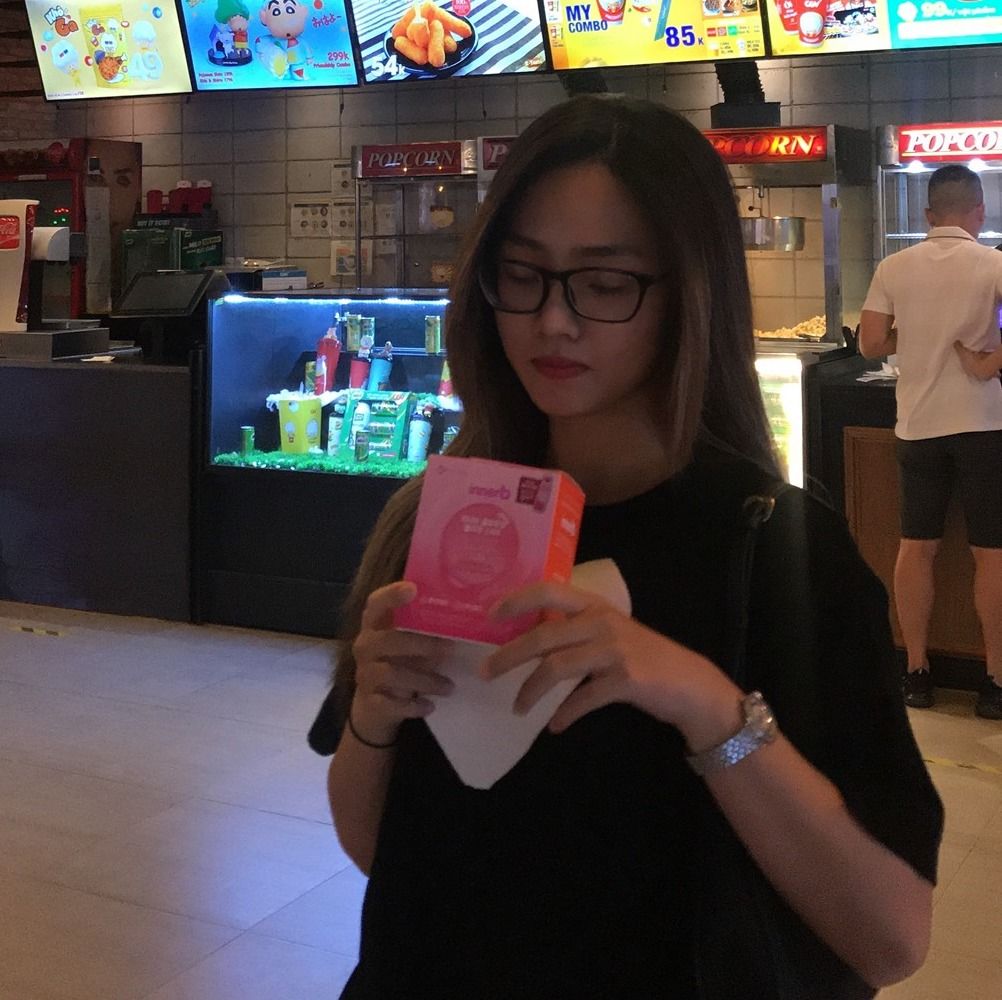
Chau Le
Cực thích đồ ngọt như sữa, kem. Thích phiêu lưu mạo hiểm nhưng vì Covid mà vô tình trở thành người chia sẻ nội dung lập trình :))
follow me :
Bài viết liên quan
Tự học Dart: Các Dart Operators (toán tử) bạn cần biết
Sep 02, 2023 • 18 min read
Flutter cơ bản: Điều cần biết khi lập trình ứng dụng đầu tiên
Aug 23, 2023 • 13 min read
Dart là gì? Giới thiệu cơ bản về ngôn ngữ lập trình Dart
Aug 21, 2023 • 11 min read
Flutter là gì? Vì sao nên học công cụ lập trình Flutter?
Aug 19, 2023 • 11 min read
Flutter cơ bản: Widget Tree, Element Tree & Render Tree
Aug 19, 2023 • 10 min read
So sánh StatelessWidget và StatefulWidget
Jul 17, 2022 • 12 min read